In this guide, we will see how we use AJAX in WordPress to submit a form and store the data in the database without reloading the page. Firstly, we will create a form where users can enter their first name, last name, and email. When they click “Submit,” the form data will be processed using AJAX and stored in the WordPress database. A success or error message will be displayed to the user dynamically.
Create the HTML Form
Start by creating a template file named formtemplate.php in your theme’s directory (e.g., wp-content/themes/your-theme/formtemplate.php
). Add the following code to create a form with basic input fields:
<?php /**Template name: Form Insert Template */ get_header(); ?> <div id="ajax-form"> <form action="" method="post" autocomplete="on"> <input type="text" id="first-name" name="first-name" placeholder="First Name" required autocomplete="given-name"> <input type="text" id="last-name" name="last-name" placeholder="Last Name" required autocomplete="family-name"> <input type="email" id="email" name="email" placeholder="Email" required autocomplete="email"> <button id="submit-button">Submit</button> </form> <div id="response-message"></div> </div> <?php get_footer();
So, after the code is saved, the Template Dropdown in the WordPress page editor will include an option labeled: “Form Insert Template”. You can select this option and the form will be displayed on the frontend.
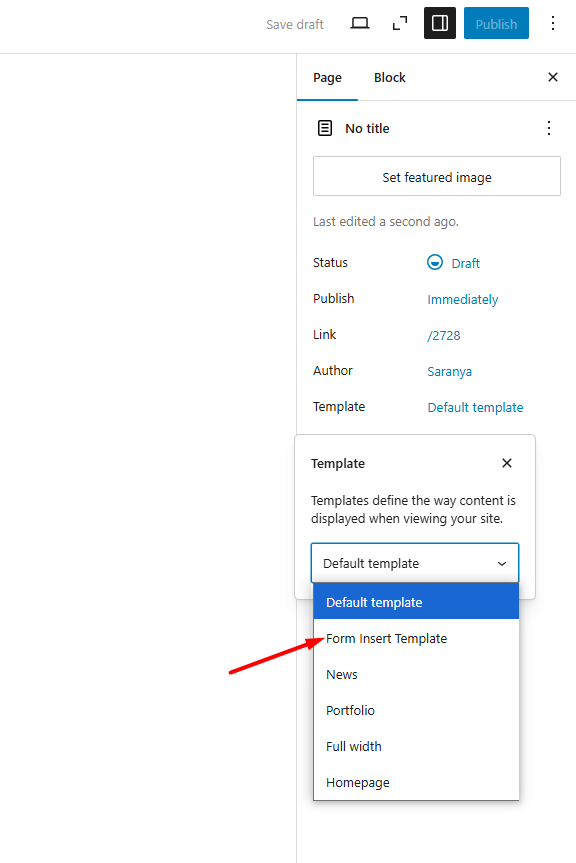
By selecting this template and publishing the page, WordPress will use the form-insert-template.php
file to render that page, displaying the form you created.
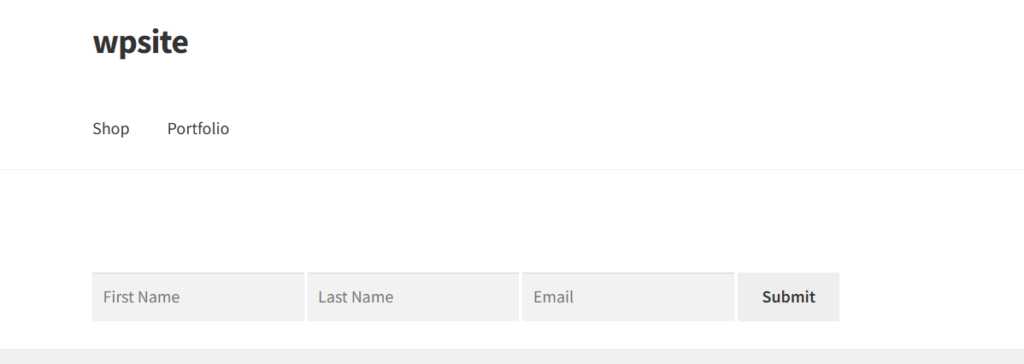
JavaScript for AJAX Requests
As a next step, let’s start how AJAX works. The following AJAX code will enable you to send the data to the server without refreshing the page. Create a new js file such as custom.js in your theme’s js folder and add the code below in it.
jQuery(document).ready(function($) { console.log(ajax_form_params.ajax_url); $('#submit-button').on('click', function(e) { e.preventDefault(); // Prevent default form submission var firstName = $('#first-name').val(); // Get the first name var lastName = $('#last-name').val(); // Get the last name var email = $('#email').val(); // Get the email // Simple validation (check if fields are empty) if (firstName === '' || lastName === '' || email === '') { $('#response-message').html('All fields are required.'); return; } // Make an Ajax request $.ajax({ url: ajax_form_params.ajax_url, // WordPress Ajax URL type: 'POST', data: { action: 'submit_form_action', // Custom action hook first_name: firstName, // Send first name last_name: lastName, // Send last name email: email // Send email }, success: function(response) { $('#response-message').html(response); // Display the success message }, error: function() { $('#response-message').html('An error occurred.'); // Display error message } }); }); });
When the user clicks the Submit button, the script:
- Gets the values entered in the form fields.
- Checks if any fields are empty.
- If all fields are filled, it sends the data to the server without reloading the page.
If the data is successfully saved, the user will see a message confirming their submission. If something goes wrong, an error message is displayed.
Enqueuing Scripts and Localizing AJAX URL
Now the code below handles the functionality of making sure that the required JavaScript files are loaded on the page for handling the AJAX request. Loads a custom JavaScript file (custom.js
) located in the assets/js/
folder of your theme. You can place both the code given below in functions.php file.
function ts_load_custom_scripts() { wp_enqueue_script('jquery'); // Ensure jQuery is loaded wp_enqueue_script('custom-ajax-script', get_template_directory_uri() . '/assets/js/custom.js', array('jquery'), null, true); // Localize the script to pass the Ajax URL wp_localize_script('custom-ajax-script', 'ajax_form_params', array( 'ajax_url' => admin_url('admin-ajax.php') )); } add_action('wp_enqueue_scripts', 'ts_load_custom_scripts');
Handling Form Submission and Storing Data in Database
The following code will handle the AJAX Requests in telling what to do when an AJAX request is made, such as processing form submissions and interacting with the created table (e.g. wp_form_submissions) in the database.
function ts_handle_form_submission() { // Check if all form fields are set and not empty if (isset($_POST['first_name']) && isset($_POST['last_name']) && isset($_POST['email'])) { global $wpdb; // Access WordPress database object $first_name = sanitize_text_field($_POST['first_name']); $last_name = sanitize_text_field($_POST['last_name']); $email = sanitize_email($_POST['email']); // Basic email validation if (!is_email($email)) { echo 'Invalid email address.'; } else { // Insert the data into the custom database table $table_name = $wpdb->prefix . 'form_submissions'; // Table name with WordPress prefix $data = array( 'first_name' => $first_name, 'last_name' => $last_name, 'email' => $email, ); $format = array('%s', '%s', '%s'); // Format for the data being inserted (strings) // Insert the data into the table $wpdb->insert($table_name, $data, $format); // Log success or errors for debugging if ($wpdb->last_error) { error_log('There was an error inserting the data: ' . $wpdb->last_error); // Log any error echo 'There was an error inserting the data.'; } else { error_log('Form data inserted successfully: ' . print_r($data, true)); // Log the inserted data echo 'Thank you, ' . $first_name . ' ' . $last_name . '! We have received your submission.'; } } } else { echo 'Please fill in all fields.'; } wp_die(); // Always call wp_die() to end the Ajax request } // Hook for logged-in users add_action('wp_ajax_submit_form_action', 'ts_handle_form_submission'); // Hook for non-logged-in users add_action('wp_ajax_nopriv_submit_form_action', 'ts_handle_form_submission');
When a user fills out the form (with fields like First Name, Last Name, Email) and clicks “Submit”. The custom JavaScript file captures the data from the form. It sends the data to the server (via the ajax_url) without refreshing the page. The user sees no page reload, and the form appears to process the information instantly. You can look for the new response in the Netwok tab where the request is sent to the server at the ajax_url
(e.g., admin-ajax.php
)
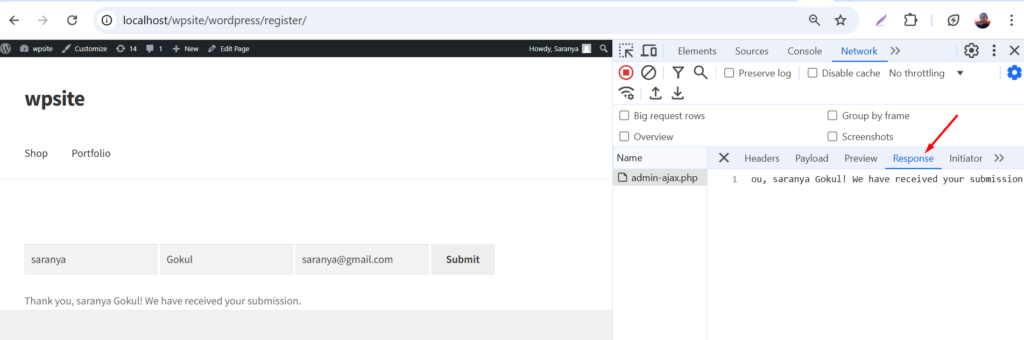
The submitted data should appear in the wp_form_submissions
table in the database.
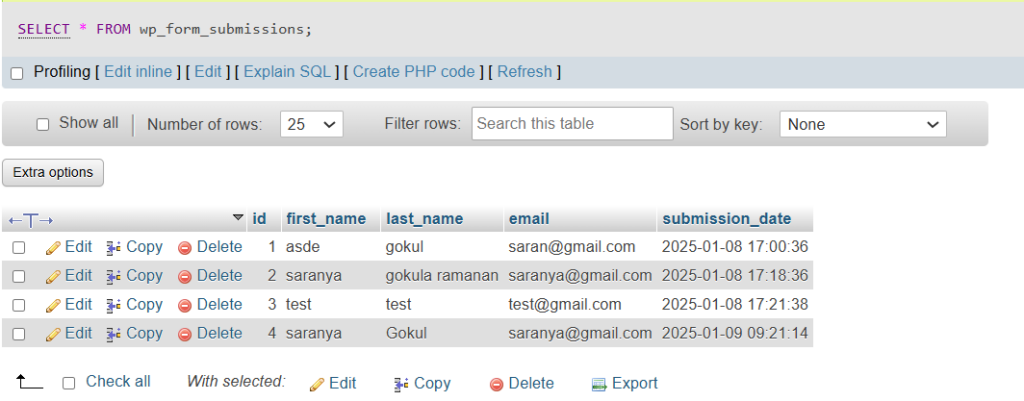
Using AJAX in WordPress can make your website more efficient and responsive, particularly for situations where you need to send and process data in real-time without reloading the page. This enhances the overall user experience by providing quick interactions.